Lifecycle hooks of the Options API
Explore the lifecycle hooks of Vue.js Options API and their relevance in managing component behavior and state.
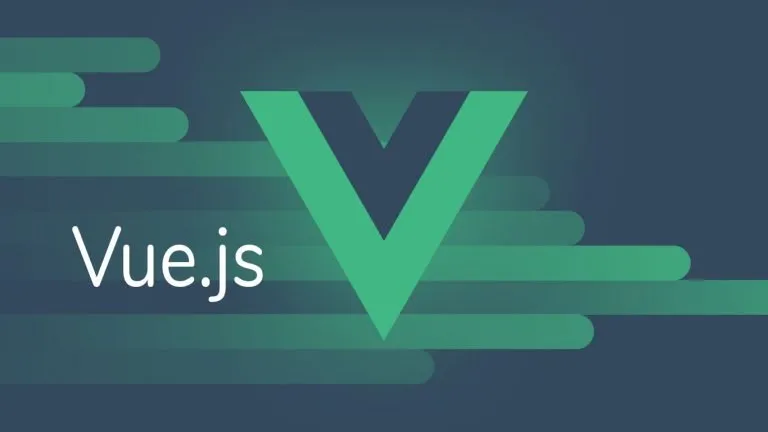
All Vue components go through the Lifecycle Hooks phases. These phases are different initialization steps. Not just the subcomponents but the main root component go through these stages. It is worth to note the purposes of these phases are different. Furthermore, Options API and Composition API lifecycle hooks differ a bit.

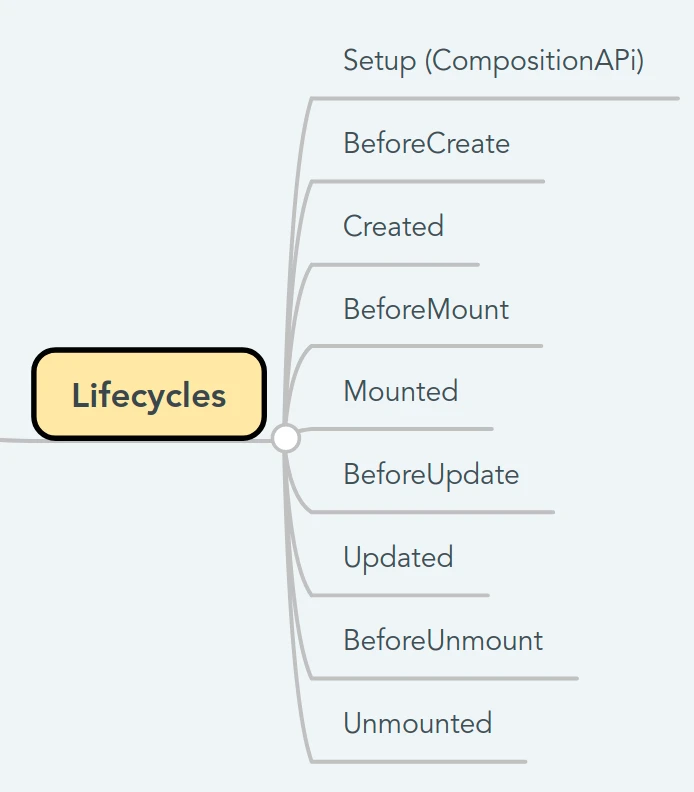
These hooks are accessible in the code of the templates as a function, and we can use these stages for our purposes. Below you see the snippet that implements all the Lifecycle Hooks for the Options API.
let vm = Vue.createApp({
data() {
return {
message: "Hello world!"
}
},
beforeCreate() {
// Before the Vue instance is initialized
console.log('beforeCreate() function called!', this.message)
},
created() {
// If we want to make changes in the data only then you can use this lifecycle
console.log('created() function called!', this.message)
},
beforeMount() {
// It is triggered when the template is compiled, but it hasn't been added to the template yet.
console.log('beforeMount() function called!', this.$el) // $el value will be the element where vue will be mounted
},
mounted() {
// This mounted lifecycle is only triggered once the template is compiled and inserted into a Document
// We can access the template here if we want to make a change in it.
console.log('mounted() function called!', this.$el)
},
beforeUpdate() {
// This hook gets called whenever an update in our data occurs but before these changes applied to the template
console.log('beforeUpdate() function called!')
},
updated() {
console.log('updated() funcction called!')
},
beforeUnmount() {
// This hook gets triggered before the instance is unmounted. Let us making last minute changes. We still have
// access to the data and methods
console.log('beforeUnmount() function called!')
},
unmounted() {
// When the instance is unmounted
console.log('unmounted() function called!')
}
})
vm.mount('#app')
Before Create
The Before Create hook runs when the component instance is initialized, before the data and computed parameter processing.
Created
The created hook runs when
- computed properties
- methods
- watchers
- reactive data
have been set up. But the mounting phase has not been started. Due to this, the "$el" DOM property is not available.
Before Mounted
Components have finished setting up their reactive state, but no DOM nodes have been created yet. (No execution in SSR approach)
Mounted
This hook will run when the component is mounted. One component is mounted when:
- all of its synchronous child components have been mounted
- it does not include the async components
- The component's DOM tree has been created and inserted into the parent container.
Before Update
It will run before any reactive property state change. We can use this hook when we want to access the DOM state before Vue updates the DOM. It is also safe to modify the component state in this hook.
"Do not mutate component state in the updated hook - this will likely lead to an infinite update loop!" - by vuejs.org
Updated
This state will run when the reactive properties have changed already.
Before Unmount
It runs before the unmount phase of a component. The component instance is still fully functional.
UnMounted
This hook runs after when the components are unmounted. One component is unmounted when all of its child components have been unmounted. Render effect and computed / watchers executions are stopped.
Conclusion & Closing
In this post, I focused on the details of the lifecycle hooks in the Options API. Sometimes, in the next VueJS-related post I am going to dive into the lifecycle hooks of the Composition API. They are a bit different but it would not be that difficult. I hope this is useful not just for me but for others too. Thanks for reading if you got here ...