[1] JIT, union types, and null-safe operator as new PHP 8 features
Deep dive into PHP 8's JIT compiler, union types, and null-safe operator with examples.
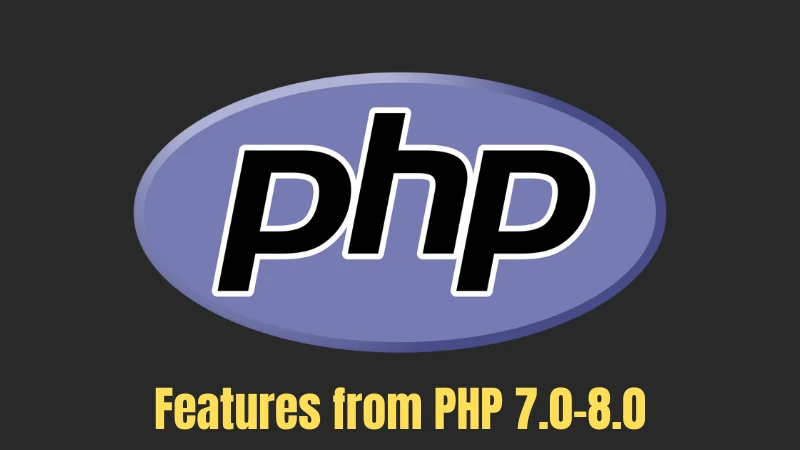
In this series, I want to go into the details of PHP8.0's New Features. PHP8.0's release date was Nov 26, 2020. Because of this, it has brought several new features into our life. JIT Compiler, Union Types, Null safe operator are just a few examples from the list. You can see the list of features here.
JIT Compiler in PHP8
JIT is a "Just In Time" compiler for PHP 8.0. The purpose of the JIT Compiler is to compile parts of the code at runtime. We could think about this as a "cached version" of the interpreted code. This code will be created at runtime. The positive effect of the compiler depends on where we want to use it. If our task contains many re-executing parts like fractal generation, then it has a huge performance boost on our application. Otherwise, it is hardly measurable.
Unfortunately, web development does not have too many re-executing code parts. So the benefit of this feature is not that relevant from the web-development point of view.
How does JIT Compiler work?
In short: The JIT compiler has a detection phase. During this process, it detects the re-executed parts of your code and marks them as "warm" or "hot" depending on the frequency. The hot parts will be compiled into an optimized machine code. This optimized code will run on the fly instead of the real code. You can read more about the topic here.
Union Types in PHP8:
class Revenue {
private int|float $revenue;
public function setRevenue(int|float $number): void {
$this->revenue = $number;
}
public function getRevenue(): int|float {
return $this->revenue;
}
}
Before PHP version 8.0, you could only declare a single type for properties, parameters, and return types. PHP 7.1 and newer versions have nullable types, which means you can declare the type to be null
with a type declaration similar to ?string
. So you can declare a type as nullable by prefixing a ?
sign to the type. For example, if a certain property can be a string
or null
, you can declare it as ?string
. With PHP 8.0 Union Types string|null
will be functionally equivalent to ?string
. You can read more here.
And lastly PHP8's Null Safe Operator
The null coalescing operator has a disadvantage. It does not work on method calls. With this feature we can do things like this:
$dateAsString = $booking->getStartDate()?->asDateTimeString();
For a bit more info you can check this link too.
Conclusion & Closing
The previous post was a retrospective about the introduced features of the previous PHP versions. Some of these features paved the way for PHP8 new features in the latest main version. The next couple of posts will focus on these features.