[1] How to start playing with Vue.js
A beginner-friendly guide to starting with Vue.js: installation setup and first steps.
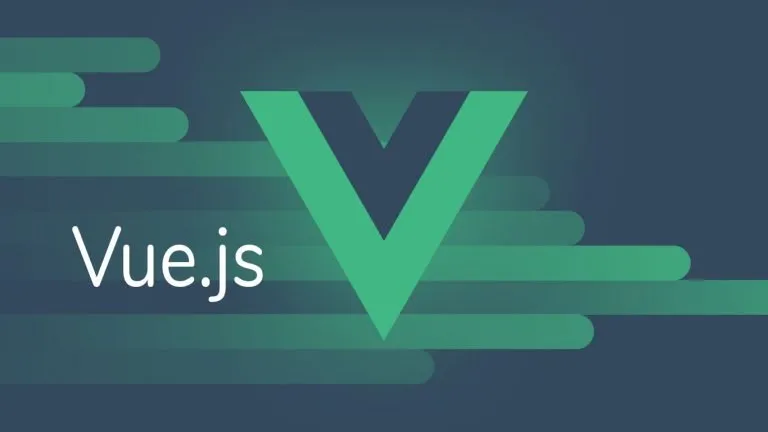
VueJS is an essential part of InertiaJS when we use the Vue adapter of Inertia. Working with this software stack, we need to know VueJS too. This note will be the first about the VueJS Framework.
Origin of VueJS
Vue was implemented in 2014 by Even You. Even used to be an Angular developer.
What is VueJS?
Vue (pronounce /VJU/) is a progressive framework for building user interfaces and client-side applications. By default, Vue components generate and manipulate HTML DOM.
How we can initialize a VueJS in the simplest way?
- Import the main Vue script in the first place
- (note: The remaining JS files should go lower than the main Vue JS.
<script src="https://unpkg.com/vue@next"></script>
<script src="app.js"></script>
- Define the main content part with an id attribute where Vue should load all the contents
<div id="app"></div>
- Define in the app.js what element it will control
Vue.createApp({}).mount('#app')
- The above line creates a Vue app with an empty object.
- We can customize Vue behavior in that object with different options
- We tell Vue which HTML element should be used as mounting point of tha app.
- This mounting point will be the root of the App if we check it in the Vue Devtools
How can we initialize a Vue App with Data?
- During the initialization Vue search for a data function for component data
- We can define here: String, Number, Boolean, Object, Array.
Vue.createApp({
data(){
return {
firstname: 'John'
}
}
}).mount('#app')
<div id="app">{{firstname}}</div>
Can we have multiple instances of the root app?
- Yes. But we can not tie it to class just to id with different names.
- Anyway, it is not a common approach to do that.
What is Vue Proxy?
- Vue has a bunch of getters and setters under the hood. These getters and setters help us to access and change data.
- Without this proxy, we should type longer code to access and change data.
- In the codes below, you can see the difference between accessing data without and with proxy lower.
vm.$data.firstname
vm.firstname
What options do we have to customize Vue App behavior?
The first parameter of the 'vue.createApp' method is an object. This object can have the following properties that define the behavior of the main app or any other component in the relevant component code. I will go through these in future posts.
- data
- props
- computed properties
- methods
- watch
- emits
- expose
Conclusion & Closing
This post is just the first step to have at least a local playground have some basic idea about Vue. The options above and other important info will come soon ...